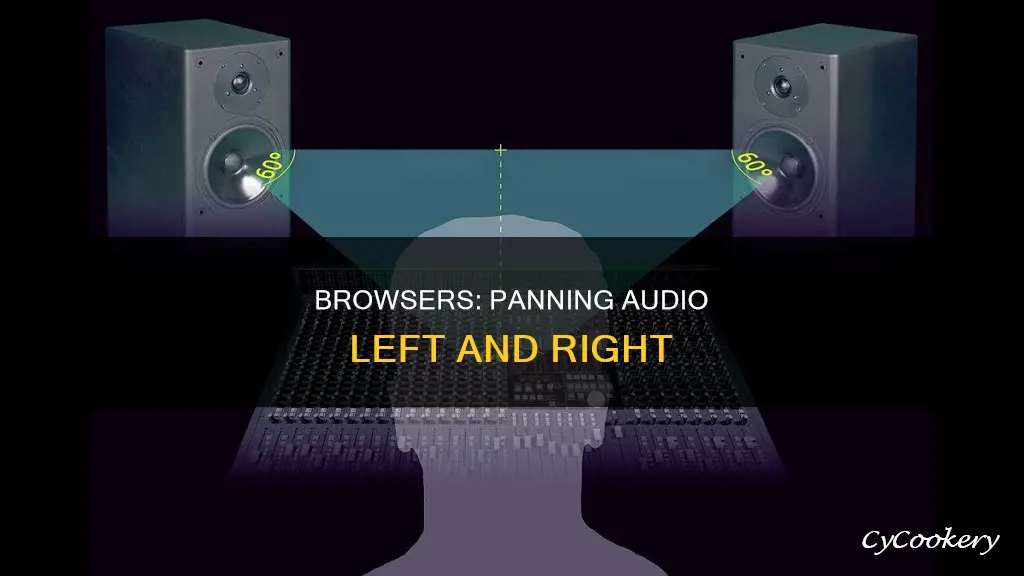
Panning audio left or right is a useful feature for multilingual events, allowing users to produce a video in two languages using stereo. There are several ways to achieve this, including using the StereoPannerNode interface of the Web Audio API, which represents a simple stereo panner node that can pan an audio stream left or right. This feature has been available across browsers since April 2021 and works on many devices and browser versions. Alternatively, there are free web tools that allow users to pan an audio file, making it play more from the left or right channel. These tools often involve selecting an audio file, dragging a slider to the desired pan position, and then downloading the modified audio file. Additionally, specific code implementations, such as those using JavaScript, can be employed to achieve audio panning in web browsers.
Characteristics | Values |
---|---|
Tools | StereoPannerNode interface of the Web Audio API, Stereo Panner, EarTrumpet, VoiceMeeter Banana |
Supported Browsers | Chrome, Firefox |
Supported Devices | Windows 10, Windows 11, iPad/Safari |
What You'll Learn
Use the StereoPannerNode interface of the Web Audio API
The StereoPannerNode interface of the Web Audio API is a simple stereo panning tool that can be used to pan an audio stream left or right. It is an AudioNode audio-processing module that positions an incoming audio stream in a stereo image using a low-cost equal-power panning algorithm.
To use the StereoPannerNode interface, you need to create a new instance of a StereoPannerNode object. This can be done using the createStereoPanner() method of the BaseAudioContext interface or by using the StereoPannerNode() constructor.
The createStereoPanner() method is the recommended way to create a StereoPannerNode. Here is an example code snippet from the MDN Web Docs:
Javascript
Const audioCtx = new AudioContext();
Const myAudio = document.querySelector("audio");
Const panControl = document.querySelector(".panning-control");
Const panValue = document.querySelector(".panning-value");
// Create a MediaElementAudioSourceNode
// Feed the HTMLMediaElement into it
Const source = audioCtx.createMediaElementSource(myAudio);
// Create a stereo panner
Const panNode = audioCtx.createStereoPanner();
// Event handler function to increase panning to the right and left
// when the slider is moved
PanControl.oninput = () => {
PanNode.pan.setValueAtTime(panControl.value, audioCtx.currentTime);
PanValue.innerHTML = panControl.value;
};
// Connect the MediaElementAudioSourceNode to the panNode
// and the panNode to the destination, so we can play the
// music and adjust the panning using the controls
Source.connect(panNode);
PanNode.connect(audioCtx.destination);
In this example, we first create an AudioContext object and select the audio element we want to control. We then create a MediaElementAudioSourceNode and a StereoPannerNode, and connect them together using the connect() method. We use an oninput event handler to change the value of the StereoPannerNode.pan parameter and update the pan value display when the slider is moved.
The StereoPannerNode() constructor is another way to create a StereoPannerNode object. Here is an example of how to use it:
Javascript
New StereoPannerNode(context, options)
The context parameter is a reference to an AudioContext object. The options parameter is an object that specifies the following options:
- A floating-point number in the range [-1,1] indicating the position of the AudioNode in the output image. The value -1 represents full left, and 1 represents full right. The default value is 0.
- An integer used to determine the number of channels used when up-mixing and down-mixing connections to any inputs to the node.
- An enumerated value describing how channels must be matched between the node's inputs and outputs.
- An enumerated value describing the meaning of the channels, which will define how audio up-mixing and down-mixing will happen. The possible values are "speakers" or "discrete".
Cleaning Hacks: Removing Black Char from Pans
You may want to see also
Try a free web tool like Stereo Panner
If you're looking to get browser audio to pan left and right, you can try a free web tool like Stereo Panner. This tool allows you to pan an audio file so that it plays more from the left or right channel. Here's a step-by-step guide on how to use Stereo Panner:
- Visit the Stereo Panner website.
- Select an audio file from your computer that you want to pan.
- Drag the slider left or right to adjust the panning of the audio.
- Click the "Submit" button to start the panning process.
- Download the audio file with your desired panning effect.
Using Stereo Panner is a simple and effective way to achieve left and right audio panning in your browser. You can experiment with different audio files and adjust the slider to achieve the desired effect.
It's worth noting that Stereo Panner is not the only tool available for this purpose. There are other web-based audio tools and software that offer similar functionality. Additionally, if you're comfortable with coding, you can explore options like the Web Audio API, which provides a StereoPannerNode interface for panning audio streams. This API offers more flexibility and customization options but may require more technical knowledge to implement.
In conclusion, Stereo Panner is a user-friendly and accessible option for anyone looking to quickly and easily pan their audio left or right without any cost or complicated setup. Give it a try and experience the impact of audio panning on your listening experience!
Panning for Gold: Techniques for Separating Fine Gold
You may want to see also
Use IXAudio2MasteringVoice::GetChannelMask to retrieve the speaker configuration
To pan audio left and right in a browser, you can use XAudio2, an audio processing API provided by Microsoft. Here's a step-by-step guide on how to use IXAudio2MasteringVoice::GetChannelMask to retrieve the speaker configuration:
- Understanding the Concept: XAudio2 is an API that allows you to manipulate audio data and create various audio effects. Panning audio involves adjusting the volume of specific channels to create the impression of sound coming from different directions.
- Initial Setup: Before using IXAudio2MasteringVoice::GetChannelMask, ensure that you have created an audio graph using XAudio2 as described in the "How to: Build a Basic Audio Processing Graph" guide. This is crucial, as attempting to initialize X3DAudio without an audio graph will result in failure.
- Retrieving the Channel Mask: To retrieve the speaker configuration, you need to call the IXAudio2MasteringVoice::GetChannelMask method. This method returns the channel mask for a specific voice. The channel mask represents the various channels in the speaker geometry reported by the audio system. Here's an example code snippet:
DWORD dwChannelMask;
PMasteringVoice->GetChannelMask(&dwChannelMask);
- Understanding the Channel Mask: The channel mask returned by IXAudio2MasteringVoice::GetChannelMask can be decoded using the SPEAKER_ positional defines declared in the X3DAUDIO.H header. For example, SPEAKER_STEREO represents the front left and front right channels, while SPEAKER_5POINT1 represents front left, front right, front centre, low frequency, back left, and back right channels.
- Speaker Configuration: By retrieving the channel mask, you can determine the speaker configuration of your audio system. This information is essential for positioning and panning audio accurately within your application.
- Panning Audio: With the knowledge of the speaker configuration, you can now adjust the volume levels of specific channels to achieve the desired panning effect. For example, to pan audio to the left, you would increase the volume of the left channels (e.g., front left, back left) while decreasing the volume of the right channels.
- Applying the Output Matrix: After calculating the desired volume levels for each channel, you need to apply the output matrix to the originating voice using IXAudio2Voice::SetOutputMatrix. This sets the volume levels for each channel, creating the panning effect between the left and right speakers.
In summary, by using IXAudio2MasteringVoice::GetChannelMask, you can retrieve the speaker configuration, which is essential for panning audio left and right. This method provides you with the channel mask, which you can then use to determine the positions of different speakers and adjust volume levels accordingly.
Potato Skin Magic for Carbon Steel Pan Seasoning
You may want to see also
Use JavaScript to pan audio of playing media
Panning audio left or right in a browser can be achieved by manipulating the stereo panning of the audio output. This can be done using JavaScript to adjust the stereo panning properties of the playing media. Here's a step-by-step guide on how to achieve this:
Step 1: HTML Setup
Firstly, you need to set up your HTML document to include an audio element that you can control with JavaScript. Add the following code to your HTML file:
Html
Your browser does not support the audio element.
Replace `"your_audio_file.mp3"` with the path to your audio file. You can also add multiple `
Step 2: JavaScript — Accessing Audio Context
For this example, create a separate JavaScript file (`your_javascript_file.js`) to keep your code organized. Start by accessing the HTML5 Audio Context in JavaScript:
Javascript
Const audioElement = document.getElementById('myAudio');
Const audioContext = new AudioContext();
Here, `audioElement` refers to the HTML `
Step 3: JavaScript — Panning Audio Left and Right
Now, you can define the `panAudioLeft()` and `panAudioRight()` functions to adjust the stereo panning:
Javascript
Function panAudioLeft() {
Const source = audioContext.createMediaElementSource(audioElement);
Const stereoPannerNode = audioContext.createStereoPanner();
Source.connect(stereoPannerNode);
StereoPannerNode.connect(audioContext.destination);
StereoPannerNode.pan.setValueAtTime(-1, audioContext.currentTime);
}
Function panAudioRight() {
Const source = audioContext.createMediaElementSource(audioElement);
Const stereoPannerNode = audioContext.createStereoPanner();
Source.connect(stereoPannerNode);
StereoPannerNode.connect(audioContext.destination);
StereoPannerNode.pan.setValueAtTime(1, audioContext.currentTime);
}
In these functions, a `StereoPannerNode` is created and connected to the audio source. The `pan` property of the `StereoPannerNode` is then set to -1 for panning left and 1 for panning right. This value determines the balance of the audio between the left and right channels.
Step 4: Test and Adjust
Now, when you load your HTML file in a browser, you should see the audio player and two buttons to pan the audio left and right. Clicking on the "Pan Left" button will pan the audio to the left speaker, and clicking on the "Pan Right" button will pan it to the right speaker.
You can adjust the panning value between -1 and 1 to fine-tune the balance of the audio. For example, a value of -0.5 would pan the audio slightly to the left, while 0 would keep it centered.
By using JavaScript to manipulate the stereo panning properties, you can dynamically control the audio output and create interesting audio effects for your web applications.
Ford C4 Pan: Retighten After Installation?
You may want to see also
Use a loopback/virtual mixer driver like VoiceMeeter Banana
VoiceMeeter Banana is an advanced audio mixer application with a virtual audio device that can be used as a virtual input/output (I/O) to mix and manage audio sources from or to any audio devices or applications. It is a powerful tool that can be used to connect and mix any audio source(s) with any audio application(s) with unparalleled control over sound quality.
VoiceMeeter Banana offers 5 inputs (3 physical and 2 virtual) and 5 outputs (3 physical and 2 virtual), as well as 5 mix buses (A1, A2, A3, B1, and B2). It supports a wide range of audio interfaces, including MME, Direct-X, KS, WaveRT, WASAPI, and ASIO. The software is compatible with Windows XP, VISTA, WIN7, WIN8, WIN8.1, and WIN10 (32/64 bits).
To install VoiceMeeter Banana, first, download the ZIP package from the VB-Audio website. Run the setup program in administrative mode and reboot your computer after installation. The package installs both the VoiceMeeter and VoiceMeeter Banana applications.
VoiceMeeter Banana is free to use with all functions available, but after 30 days, you will be invited to purchase a license or continue evaluating the program. The application does not require an internet connection and does not collect any user data.
With VoiceMeeter Banana, you can mix your voice and video game audio on Skype, use multiple USB headsets on a computer, play video games in 5.1 while keeping the microphone for communication, and much more. It also offers features such as parametric EQ, audio recording, 3D panoramic control, and audio effects.
How to Prevent Steak from Sticking to Your Pan
You may want to see also
Frequently asked questions
You can use a third-party app such as VoiceMeeter Banana, which is a loopback/virtual mixer driver.
You can use the StereoPannerNode interface of the Web Audio API. This feature has been available across browsers since April 2021 and works with a simple pan property that takes a value between -1 (full left pan) and 1 (full right pan).
You can use a free web tool like Audioalter, which allows you to select an audio file and then drag a slider left or right to pan the audio accordingly.