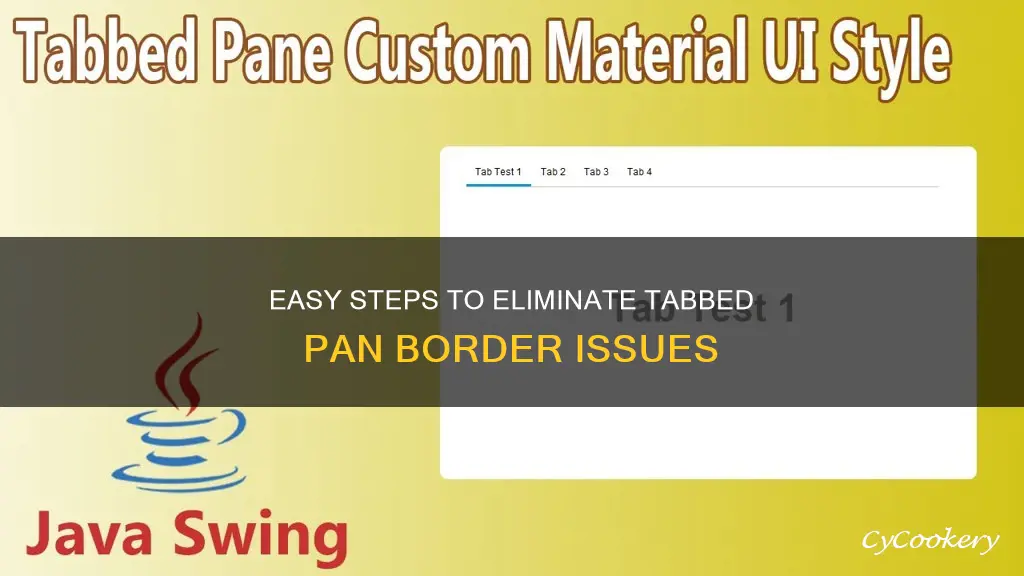
Removing tabbed pan borders is a simple process that can be achieved in a few different ways depending on the programming language and framework being used. For instance, in Java, one can use the JTabbedPane class to manage tabs and their respective borders. By utilising the remove(int index) method, one can remove a tab at a specified index, thereby eliminating its border. Alternatively, the remove(Component component) method targets a specific child component to remove its associated tab and border. If the objective is to remove all tabs and their borders, the removeAll() method is available. Similar methods can be applied to other frameworks, such as Bootstrap, where CSS styling can be used to manipulate borders and padding.
Characteristics | Values |
---|---|
How to remove a tab from a tab pane | Use the .remove(index) method of JTabbedPane |
How to disable a tab in a JTabbedPane Container | Use the setEnabledAt() method and set it to false with the index of the tab you want to disable |
Remove all tabs | Use the removeAll() method |
Remove a tab with a specified child component | Use the remove(Component component) method |
What You'll Learn
Removing tab with a specific index
Removing a tab with a specific index can be achieved using the remove(index) method of the JTabbedPane class. This method allows you to specify the index of the tab you want to remove, providing a straightforward way to eliminate a particular tab from the tabbed pane.
Here's an example code snippet demonstrating how to use the `remove(index)` method:
Java
Import javax.swing.*;
Import java.awt.*;
Public class TabbedPaneRemoveTab extends JPanel {
Public TabbedPaneRemoveTab() {
InitializeUI();
}
Public static void showFrame() {
JPanel panel = new TabbedPaneRemoveTab();
Panel.setOpaque(true);
JFrame frame = new JFrame("JTabbedPane Demo");
Frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
Frame.setContentPane(panel);
Frame.pack();
Frame.setVisible(true);
}
Public static void main(String[] args) {
SwingUtilities.invokeLater(TabbedPaneRemoveTab::showFrame);
}
Private void initializeUI() {
Final JTabbedPane pane = new JTabbedPane(JTabbedPane.LEFT);
Pane.addTab("A Tab", new JPanel());
Pane.addTab("B Tab", new JPanel());
JPanel tabPanel = new JPanel();
Pane.addTab("C Tab", tabPanel);
Pane.addTab("D Tab", new JPanel());
Pane.addTab("E Tab", new JPanel());
// Remove a specific tab by specifying its index
Pane.remove(2); // This will remove the tab at index 2 ("C Tab")
JButton button = new JButton("Remove All Tabs");
Button.addActionListener(e -> {
// Remove all tabs from JTabbedPane
Pane.removeAll();
});
This.setLayout(new BorderLayout());
This.setPreferredSize(new Dimension(500, 200));
This.add(pane, BorderLayout.CENTER);
This.add(button, BorderLayout.SOUTH);
}
}
In the above code, the `remove(2)` statement targets the tab at index 2, which is "C Tab" in this case. This statement will remove that specific tab from the tabbed pane.
It's important to note that the tab indices start from 0, so "A Tab" has an index of 0, "B Tab" has an index of 1, and so on.
Additionally, you can also remove a tab by specifying its title using the removeTabAt(title) method. This method iterates through the tabs and removes the first tab with a matching title.
Here's an example of how to use the `removeTabAt(title)` method:
Java
Private void removeTabWithTitle(String tabTitleToRemove) {
For (int i = 0; i < tabbedPane.getTabCount(); i++) {
String tabTitle = tabbedPane.getTitleAt(i);
If (tabTitle.equals(tabTitleToRemove)) {
TabbedPane.remove(i);
Break;
}
}
}
In this code, the `removeTabWithTitle` method takes the `tabTitleToRemove` as a parameter and iterates through the tabs in the tabbed pane. When it finds a tab with a matching title, it removes that tab using the `remove(i)` method, where `i` is the index of the tab with the matching title.
By utilizing these methods, you can effectively remove tabs with specific indices or titles from a tabbed pane in your Java application.
Dutch Oven vs. Cast Iron: What's the Difference?
You may want to see also
Removing tab with a specific child component
To remove a tab with a specific child component, you can use the remove(Component component) method. This method allows you to specify the child component that you want to remove from the tabbed pane. Here's an example code snippet demonstrating how to use this method:
Java
Import javax.swing.*;
Import java.awt.;
Public class TabbedPaneRemoveTab extends JPanel {
Public TabbedPaneRemoveTab() {
InitializeUI();
}
Public static void showFrame() {
JPanel panel = new TabbedPaneRemoveTab();
Panel.setOpaque(true);
JFrame frame = new JFrame("JTabbedPane Demo");
Frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
Frame.setContentPane(panel);
Frame.pack();
Frame.setVisible(true);
}
Public static void main(String[] args) {
SwingUtilities.invokeLater(TabbedPaneRemoveTab::showFrame);
}
Private void initializeUI() {
Final JTabbedPane pane = new JTabbedPane(JTabbedPane.LEFT);
Pane.addTab("A Tab", new JPanel());
Pane.addTab("B Tab", new JPanel());
// Create a panel with a specific name, e.g., "tabPanel"
JPanel tabPanel = new JPanel();
Pane.addTab("C Tab", tabPanel);
Pane.addTab("D Tab", new JPanel());
Pane.addTab("E Tab", new JPanel());
// Remove the tab that contains the specific child component "tabPanel"
Pane.remove(tabPanel);
JButton button = new JButton("Remove All Tabs");
Button.addActionListener(e -> {
// Remove all tabs from the JTabbedPane
Pane.removeAll();
});
This.setLayout(new BorderLayout());
This.setPreferredSize(new Dimension(500, 200);
This.add(pane, BorderLayout.CENTER);
This.add(button, BorderLayout.SOUTH);
}
}
In the above code, we create a `JTabbedPane` with multiple tabs. Then, we create a `JPanel` with the name "tabPanel" and add it as a tab to the `JTabbedPane". To remove the tab with the specific child component "tabPanel", we use the `remove(Component component)` method, passing "tabPanel" as the argument. This will remove the tab that contains the specified child component.
You can also use other methods to remove tabs from a `JTabbedPane`, such as the remove(int index) method to remove a tab at a specified index, or the removeTabAt(int index) method, which is another way to remove a tab by specifying its index. Additionally, the removeAll() method can be used to remove all tabs from the `JTabbedPane`.
Pan-Seared Filet Mignon: Restaurant Quality at Home
You may want to see also
Removing all tabs
To remove all tabs in Google Docs, you must first display the ruler by going to the View menu and selecting "Show Ruler". This will place a checkmark next to it and display the ruler around your document.
Once the ruler is visible, you can remove all the tab stops by selecting the indicators on the ruler and dragging them downward out of the ruler. If you remove all the tab stops, Google Docs will return to the default setting of moving the cursor by 0.5 inches when you press the tab key.
In Java, you can remove all tabs from a JTabbedPane by using the removeAll() method. Here is an example code snippet that demonstrates this:
Java
JButton button = new JButton("Remove All Tabs");
Button.addActionListener(e -> {
// Remove all tabs from JTabbedPane
Pane.removeAll();
});
Cover Foil Roasting Pan: Quick Tips
You may want to see also
Disabling a tab
Java
In Java, if you are using the `JTabbedPane` class to manage your tabs, you can remove a specific tab by using the `remove(int index) method, where `index` is the position of the tab you want to remove. For example:
Java
Pane.remove(pane.getTabCount() - 1);
You can also remove a tab by specifying the child component:
Java
Pane.remove(tabPanel);
To remove all tabs at once, you can use the `removeAll()` method:
Java
Pane.removeAll();
Bootstrap
If you are using Bootstrap, you can disable a tab by removing the `data-toggle="tab"` attribute from the `a` element that represents the tab. This will make the tab non-toggleable, and clicking on it will have no effect. Here's an example:
Html
Additionally, you can visually indicate that a tab is disabled by adding the `disabled` class to the parent `li` element:
Html
Combining both methods will result in a completely disabled tab:
Html
Other Approaches
In some cases, you may want to prevent the tab from being clicked while still allowing it to be toggleable. This can be achieved by adding custom JavaScript to handle the click event and prevent the default behaviour. Here's an example:
Javascript
$(".nav-tabs a[data-toggle=tab]").on("click", function(e) {
If ($(this).hasClass("disabled")) {
E.preventDefault();
Return false;
}
});
This JavaScript code checks if the tab has the "disabled" class and, if so, prevents the default click behaviour, effectively disabling the tab.
It's important to note that the specific implementation may vary depending on the programming language, framework, and your specific use case. Always refer to the official documentation and resources for the tools you are using.
GMAT Test Prep: Do You Need a Tutor?
You may want to see also
Removing tab border and padding
Removing tab borders and padding can be achieved through various methods, depending on the programming language or software being used. Here are some common approaches:
HTML and CSS:
By modifying the CSS styles associated with the tab elements, you can remove borders and adjust padding. For example, you can use properties like "border: none" or "padding: 0" to remove borders and padding, respectively. Additionally, you can target specific tab states, such as the active or selected tab, by using CSS pseudo-classes like ":hover", ":active", or ".selected".
Here's an example code snippet demonstrating how to remove the bottom border from the active tab in CSS:
Css
Ul#tabs li a.selected {
Color: #000; background-color: #f1f0ee; font-weight: bold; padding: 0.3em 0.3em 0.38em 0.3em;
Border-bottom: none; /* Remove the bottom border */
}
Java:
When working with Java, you can utilise the JTabbedPane class to manage tabs and their content. To remove a specific tab, you can use the remove(int index) method, where `index` is the position of the tab you want to remove. Alternatively, you can use remove(Component component) to remove a tab by specifying its child component. If you want to remove all tabs at once, you can use the removeAll() method.
Here's an example of how to remove a tab at a specific index in Java:
Java
Pane.remove(pane.getTabCount() - 1); // Remove the last tab
Chrome and Windows:
If you're encountering issues with Chrome's tab borders when using the Windows split-screen feature (Win + ←), there are a few workarounds. One solution is to use third-party software like Virtual Display Manager (VDM) or DisplayFusion (PRO version) to achieve true maximisation of windows to virtual monitors, eliminating the border issue. Alternatively, you can try using Firefox, which handles maximisation differently and allows tab clicking from the very top edge of the screen.
Pan-Seared Top Sirloin: A Quick Steak Dinner
You may want to see also
Frequently asked questions
You can use the .remove(index) method of JTabbedPane to remove a tab at a specified index.
You can use the .remove(Component component) method to remove specific tabs by specifying their child component.
You can use the .removeAll() method to remove all tabs from a tabbed pane.
To disable a tab, use the setEnabledAt() method and set it to false with the index of the tab you want to disable.