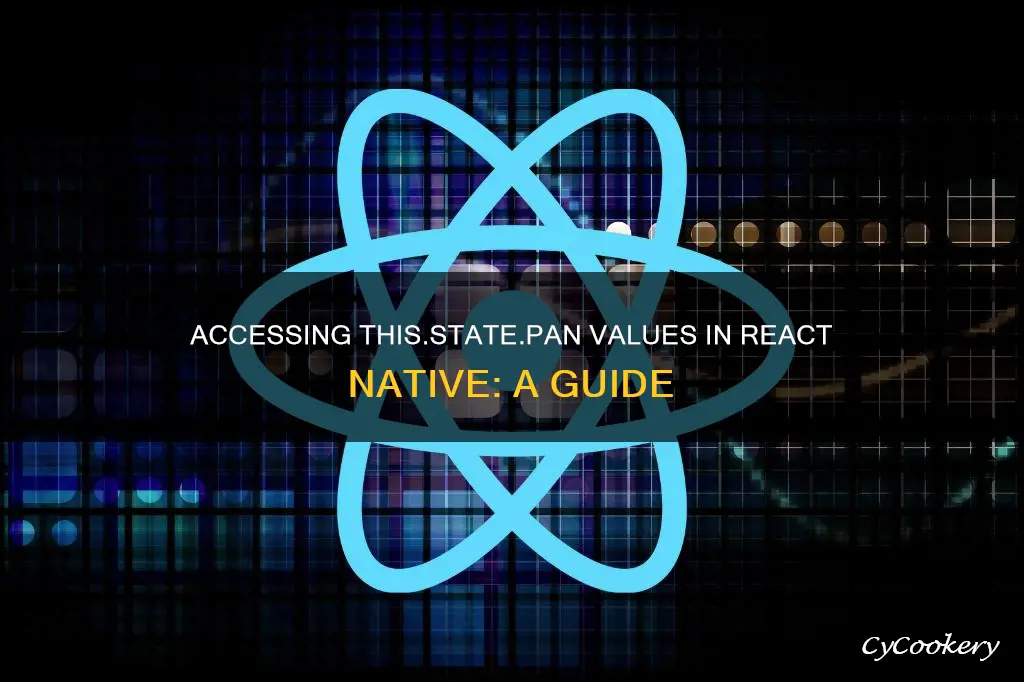
React Native has two types of data that control a component: props and state. Props are set by the parent and remain fixed throughout the lifetime of a component, while state is used for data that will change. State is typically initialised in the constructor, and then the setState function is used to change it. To access the state values, use the this.state.propertyname syntax.
Characteristics | Values |
---|---|
How to get this.state.pan values in React Native | Use the this.state.propertyname syntax to refer to the state object anywhere in the component |
Where to get this.state.pan values in React Native | Refer to the state object in the render() method |
How to change this.state.pan values in React Native | To change a value in the state object, use the this.setState() method |
When to use this.state.pan values in React Native | Use this.state.pan values when a value in the state object changes |
What You'll Learn
How to create a state object in React Native
React components have a built-in state object where you store property values that belong to the component. When the state object changes, the component re-renders.
To create a state object in React Native, you need to initialize the state in the constructor method. Here's an example code snippet demonstrating this:
Javascript
Class Car extends React.Component {
Constructor(props) {
Super(props);
This.state = {
Brand: "Ford",
Model: "Mustang",
Color: "red",
Year: 1964,
};
}
Render() {
Return (
My {this.state.brand}
It is a {this.state.color} {this.state.model} from {this.state.year}.
}
}
In the above code, the `state` object is initialized within the `constructor` method. It contains properties such as `brand`, `model`, `color`, and `year`, which are specific to the `Car` component.
You can access the values of the state object anywhere within the component using the `this.state.propertyname` syntax. For example, in the `render` method, the values of `brand`, `model`, `color`, and `year` are dynamically inserted into the JSX using `this.state.brand`, `this.state.color`, and so on.
Remember that state objects are used for data that is expected to change. For fixed data, you would use `props` instead. Additionally, while objects in state can be modified directly, it is recommended to treat them as immutable and always create a new object or copy when making updates.
Unraveling the Mystery: Identifying Your Pan's True Nature
You may want to see also
How to use the state object in React Native
React components have a built-in state object where you store property values that belong to the component. The state object is initialized in the constructor and can contain as many properties as you like.
Here's an example:
Class Car extends React.Component {
Constructor(props) {
Super(props);
This.state = {
Brand: "Ford",
Model: "Mustang",
Color: "red",
Year: 1964
};
}
Render() {
Return (
My {this.state.brand}
It is a {this.state.color} {this.state.model} from {this.state.year}.
;
}
}
In the above code, the state object contains four properties: brand, model, color, and year. You can refer to the state object anywhere in the component using the `this.state.propertyname` syntax.
To change a value in the state object, use the `this.setState()` method. For example, let's say you want to change the color property:
Class Car extends React.Component {
Constructor(props) {
Super(props);
This.state = {
Brand: "Ford",
Model: "Mustang",
Color: "red",
Year: 1964
};
}
ChangeColor = () => {
This.setState({ color: "blue" });
}
Render() {
Return (
My {this.state.brand}
It is a {this.state.color} {this.state.model} from {this.state.year}.
;
}
}
In the above code, the `changeColor` function is called when the "Change color" button is clicked. This function uses the `this.setState()` method to update the color property of the state object to "blue".
It is important to note that you should always use the `setState()` method to change the state object. This ensures that the component knows it has been updated and triggers a re-render.
Additionally, state can be updated in response to event handlers, server responses, or prop changes. State can hold any kind of JavaScript value, including objects. However, you should not change objects that you hold in the React state directly. Instead, when you want to update an object, create a new one or make a copy of an existing one, and then set the state to use that copy.
Cast Iron Revolution: The Ultimate Hot Pot Experience
You may want to see also
How to change the state object in React Native
React components have a built-in state object, which is where you store property values that belong to the component. To change a value in the state object, use the this.setState() method.
Class Car extends React.Component {
Constructor(props) {
Super(props);
This.state = {
Brand: "Ford",
Model: "Mustang",
Color: "red",
Year: 1964,
};
}
ChangeColor = () => {
This.setState({ color: "blue" });
}
Render() {
Return (
My {this.state.brand}
It is a {this.state.color} {this.state.model} from {this.state.year}.
;
}
}
In the above example, the state object contains information about a car, including its brand, model, color, and year. The `changeColor` function is called when the "Change color" button is clicked, and it updates the color property of the state object to "blue". This will trigger a re-render of the component, and the updated color will be displayed.
It is important to note that you should always use the `setState()` method to change the state object. This ensures that the component knows it has been updated and calls the render() method, as well as any other lifecycle methods.
Additionally, state updates may be asynchronous, so it is important not to rely on the current values of `this.props` and `this.state` when calculating the next state. Instead, use the second form of `setState()` that accepts a function rather than an object. This function will receive the previous state as the first argument and the props at the time the update is applied as the second argument.
Butter Spray: Harmful to Non-Stick Pans?
You may want to see also
How to import useState from 'react' in React Native
To import `useState` from React in React Native, you need to follow these steps:
Firstly, import the `useState` hook at the top of your component file:
Javascript
Import { useState } from 'react';
Then, you can declare one or more state variables using `useState` inside your functional component:
Javascript
Function MyComponent() {
Const [age, setAge] = useState(28);
Const [name, setName] = useState('Taylor');
}
In the above example, `age` and `name` are state variables, and `setAge` and `setName` are the functions used to update their respective state values. The values in the `useState` calls, `28` and `'Taylor'`, represent the initial state for each variable.
The `useState` hook is used to add state variables to your component and allows you to "remember" a value within a component function. It returns an array containing the current state and a function to update that state.
You can then use these state variables and update functions throughout your component as needed. For example:
Javascript
Function handleClick() {
SetAge(age + 1);
}
In this example, the `handleClick` function increments the `age` state variable by 1 when called.
Mala Hot Pot: A Healthy Indulgence or a Dietary Disaster?
You may want to see also
How to use props in React Native
Props are a fundamental concept in React Native, which are used to customize and configure components. They are essentially parameters or attributes that are passed to a component when it is created, allowing for flexibility and reusability. Here's a comprehensive guide on how to use props effectively in React Native:
Understanding Props
Props, short for properties, are inputs that are passed to a component. They are used to customize the behaviour and appearance of a component. In React Native, components can be customized with different parameters, and these parameters are called props. For example, the Image component in React Native has a prop called "source" that determines which image is displayed.
Using Props in React Native
To use props in React Native, follow these steps:
- Define the Props: Determine what information or configuration your component needs to function correctly. For example, if you're creating a "Greeting" component, you might need a prop for the person's name.
- Pass the Props: When using the component in your code, pass the necessary props to it. For example, if you have a "Greeting" component, you can use the "name" prop to customize the greeting for each person.
- Access the Props: Inside the component, you can access the passed props using "this.props". For example, in a functional component, you can use "props.name" to access the value of the "name" prop.
- Utilize the Props: Use the passed props within the component to customize its behaviour or appearance. For example, you can display the value of the "name" prop in the UI to greet the person by name.
Best Practices and Recommendations
- Reusable Components: Design your components to be reusable across different parts of your application. Props allow you to customize components, making them versatile and reducing code duplication.
- Naming Conventions: Follow consistent naming conventions for your props. Use descriptive names that clearly indicate the purpose of the prop. Avoid using generic names like "data" or "value".
- Avoid Mutating Props: In React, components must act like pure functions with respect to their props. This means that you should not modify or mutate the props directly. Instead, use the props to determine the component's output or behaviour.
- Container and Presentational Components: Separate your components into container components and presentational components. Container components are responsible for handling state and passing data to presentational components through props. Presentational components focus solely on the visual presentation of the data and do not maintain their own state.
- Use Default Props: You can define default values for your props using the "defaultProps" object. This allows you to provide fallback values in case a prop is not provided when the component is used.
- Prop Types: Utilize prop types to validate the data types of the props passed to your components. This helps catch potential type-related errors early and ensures that your components receive the expected types of data.
By following these guidelines and understanding the fundamentals of props in React Native, you can effectively leverage them to build dynamic, customizable, and reusable components for your applications.
The Many Sizes of Lodge Cast Iron Pans: A Comprehensive Guide
You may want to see also
Frequently asked questions
To get this.state.pan values in React Native, you need to refer to the state object by using the this.state.propertyname syntax.
You can refer to the state object anywhere in the component, including in the render() method.
To change the value of this.state.pan, use the this.setState() method. For example, this.setState({pan: "new value"}) will change the value of this.state.pan to "new value".
When you change the value of this.state.pan, the component will re-render, meaning that the output will change according to the new value.