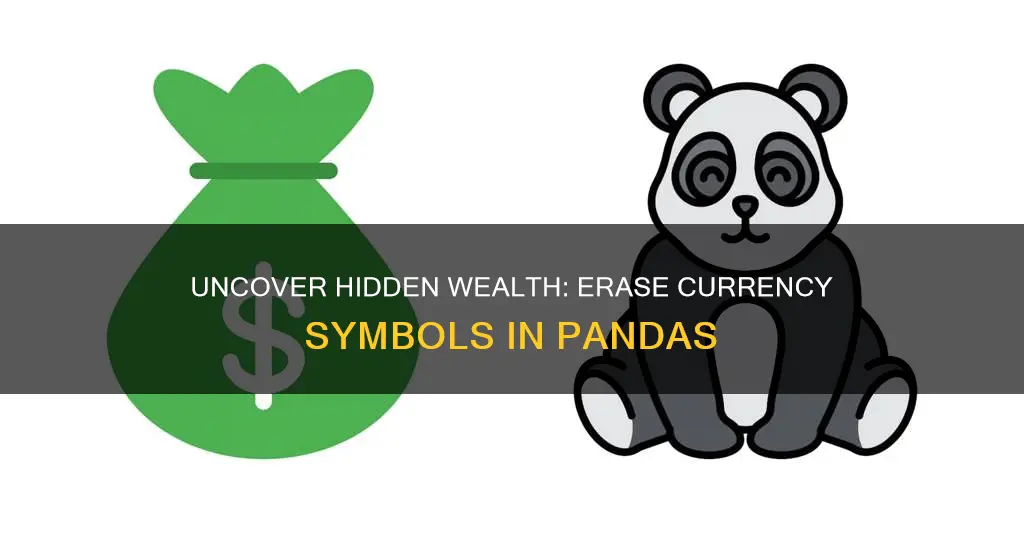
There are several ways to remove currency symbols from a Pandas dataframe. One way is to use the replace() function with regular expressions enabled:
python
df['column_name'] = df['column_name'].replace({'\$': '', ',': ''}, regex=True)
Alternatively, you can use the str.replace() function:
python
df['column_name'] = df['column_name'].str.replace('\$', '', regex=True)
If you want to remove the currency symbol from the entire dataframe, you can use the following code:
python
colstocheck = dftest.columns
dftest[colstocheck] = dftest[colstocheck].replace({'\$': ''}, regex=True)
Make sure to escape the dollar sign with a backslash when using regular expressions.
What You'll Learn
- Use the .replace() function to remove the dollar sign from each row
- Use the str.replace() function to remove the dollar sign from a column
- Use the apply() function with a custom function to remove the dollar sign from a column
- Use the applymap() function with a lambda function to remove the dollar sign from the entire dataframe
- Use the str.strip() function to remove the dollar sign from a column
Use the .replace() function to remove the dollar sign from each row
To remove the dollar sign from each row in a Pandas dataframe, you can use the `.replace()` function. This function allows you to replace specific values in a dataframe with other values. In this case, you want to replace the dollar sign with nothing, effectively removing it from the data. Here's an example of how you can use the `.replace()` function to achieve this:
Python
Import pandas as pd
Sample dataframe with dollar signs in the 'Price' column
Df = pd.DataFrame({'Price': ['$495,000', '$500,000', '$480,500']})
Using .replace() to remove dollar signs from the 'Price' column
Df['Price'] = df['Price'].str.replace('$', '')
Print(df)
Running this code will output a dataframe with the dollar signs removed from the 'Price' column:
Price
0 495,000
1 500,000
2 480,500
Note that the dollar sign is removed, but the commas remain. If you also want to remove the commas and convert the values to numeric format, you can extend the code as follows:
Python
Import pandas as pd
Sample dataframe with dollar signs in the 'Price' column
Df = pd.DataFrame({'Price': ['$495,000', '$500,000', '$480,500']})
Using .replace() to remove dollar signs and commas from the 'Price' column
Df['Price'] = df['Price'].str.replace('$', '').str.replace(',', '').astype(float)
Print(df)
This code will output a dataframe with the dollar signs and commas removed, and the values converted to floats:
Price
0 495000.0
1 500000.0
2 480500.0
Additionally, if you have dollar signs in multiple columns of your dataframe, you can specify the columns you want to modify by providing a list of column names:
Python
Import pandas as pd
Sample dataframe with dollar signs in multiple columns
Df = pd.DataFrame({'Price': ['$495,000', '$500,000', '$480,500'], 'Amount': ['$100', '$200', '$300']})
Columns with dollar signs
Columns_to_replace = ['Price', 'Amount']
Using .replace() to remove dollar signs from the specified columns
Df [columns_to_replace] = df [columns_to_replace].replace('$', '', regex=True)
Print(df)
This code will output a dataframe with the dollar signs removed from both the 'Price' and 'Amount' columns:
Price Amount
0 495,000 100
1 500,000 200
2 480,500 300
The Chongqing Hot Pot Bunker Phenomenon: Exploring the Underground Dining Experience
You may want to see also
Use the str.replace() function to remove the dollar sign from a column
To remove the dollar sign from a column in pandas, you can use the str.replace() function. This function allows you to replace specific characters or substrings in a string with another character or substring. In this case, you would use it to replace the dollar sign with an empty string, effectively removing it from the column values.
Python
Import pandas as pd
Sample data
Data = {"price": ["$495,000", "$500,000"]}
Df = pd.DataFrame(data)
Remove dollar sign using str.replace()
Df['price'] = df['price'].str.replace('$', '')
Print(df)
In this example, the original dataframe `df` has a column called "price" with dollar signs in each value. By using the str.replace() function, we replace the dollar sign (`'$'`) with an empty string (`''`), effectively removing it from the column values. The resulting dataframe will have the same column "price" but without the dollar signs.
You can also use the replace() function without the `str` accessor, as shown in the following example:
Python
Import pandas as pd
Sample data
Data = {"price": ["$495,000", "$500,000"]}
Df = pd.DataFrame(data)
Remove dollar sign using replace()
Df = df.replace('$', '', regex=True)
Print(df)
In this example, we use the replace() function directly on the dataframe `df`. We specify `'$'` as the value to be replaced and `''` as the replacement value. By setting regex=True, we indicate that we want to perform a regular expression replacement, which allows us to replace all occurrences of the dollar sign in the column.
Both approaches will achieve the same result of removing the dollar sign from the column values. You can choose the one that you find more readable or convenient for your specific use case.
The Secret to Taiwanese Hot Pot Sauce: A Step-by-Step Guide
You may want to see also
Use the apply() function with a custom function to remove the dollar sign from a column
To remove the dollar sign from a column in Pandas, you can use the apply() function with a custom function. Here's an example code snippet:
Python
Import pandas as pd
Sample data
Data = {"dollars": ["$495,000", "$500,000"]}
Df = pd.DataFrame(data)
Custom function to remove dollar sign
Def remove_dollar_sign(x):
If isinstance(x, str):
Return x.replace('$', '')
Else:
Return x
Apply the function to the column
Df['dollars'] = df['dollars'].apply(remove_dollar_sign)
Print the updated dataframe
Print(df)
In this code, we first import the Pandas library and create a sample dataframe with a column named "dollars" containing dollar amounts with the dollar sign.
Next, we define a custom function `remove_dollar_sign` that takes a value `x` as input. We use the `isinstance()` function to check if `x` is a string. If it is a string, we use the `replace()` method to remove the dollar sign and return the updated value. Otherwise, we return the original value.
We then use the `apply()` function to apply the `remove_dollar_sign` function to each value in the "dollars" column of the dataframe. This will replace the dollar sign in each value of the column.
Finally, we print the updated dataframe, which will now have the dollar signs removed from the "dollars" column.
This approach allows you to create a custom function that specifically targets the removal of the dollar sign, and then use the `apply()` function to apply that function to the entire column. This is a flexible way to clean and transform data in Pandas dataframes.
Rubber Muffin Pans: Grease or Not?
You may want to see also
Use the applymap() function with a lambda function to remove the dollar sign from the entire dataframe
The `applymap()` method in pandas allows you to apply a function to every element of a DataFrame. This can be used to remove dollar signs from a column or the entire DataFrame.
Here's an example of how to use the `applymap()` function with a lambda function to remove the dollar sign from the entire DataFrame:
Python
Import pandas as pd
Sample DataFrame with dollar signs
Df = pd.DataFrame({"dollars": ["$495,000", "$500,000"]})
Using applymap() with a lambda function to remove dollar signs
Df['dollars'] = df['dollars'].applymap(lambda x: x.replace('$', ''))
Print(df)
In this code, we first import the pandas library and create a sample DataFrame called `df` with a column called "dollars" containing values with dollar signs.
Next, we use the `applymap()` function on the "dollars" column and pass a lambda function as an argument. The lambda function `lambda x: x.replace('$', '')` takes each element `x` in the column and replaces the dollar sign with an empty string, effectively removing the dollar sign.
Finally, we print the updated DataFrame `df`, which should now contain the values without the dollar signs.
You can also use the `replace()` function or the `str.replace()` method directly on the column to remove the dollar signs, as shown in some of the sources. However, the `applymap()` function with a lambda function provides a flexible and concise way to achieve this.
Easy Ways to Remove Stickiness from Your Pans
You may want to see also
Use the str.strip() function to remove the dollar sign from a column
To remove the dollar sign from a column in pandas, you can use the str.strip() function. This function removes specified characters from a string, and in this case, you can use it to remove the dollar sign. Here's an example of how you can use it:
Python
Import pandas as pd
Create a sample dataframe
Data = {"dollars": ["$495,000", "$500,000"]}
Df = pd.DataFrame(data)
Remove the dollar sign using str.strip()
Df['dollars'] = df['dollars'].str.strip('$')
Print the updated dataframe
Print(df)
In this code, we first import the pandas library and create a sample dataframe with a column called "dollars" containing dollar amounts with the dollar sign. Then, we use the str.strip() function to remove the dollar sign from the "dollars" column and assign the result back to the same column. Finally, we print the updated dataframe, which should now have the dollar sign removed from the "dollars" column.
You can also use the str.replace() function to achieve the same result:
Python
Import pandas as pd
Create a sample dataframe
Data = {"dollars": ["$495,000", "$500,000"]}
Df = pd.DataFrame(data)
Remove the dollar sign using str.replace()
Df['dollars'] = df['dollars'].str.replace('$', '')
Print the updated dataframe
Print(df)
In this code, we use the str.replace() function to replace the dollar sign with an empty string, effectively removing it from the "dollars" column.
Additionally, if you want to remove both the dollar sign and the comma from the column, you can use the following code:
Python
Import pandas as pd
Create a sample dataframe
Data = {"dollars": ["$495,000", "$500,000"]}
Df = pd.DataFrame(data)
Remove the dollar sign and comma using str.replace()
Df['dollars'] = df['dollars'].str.replace('$', '').str.replace(',', '')
Print the updated dataframe
Print(df)
In this code, we use two str.replace() functions consecutively to first remove the dollar sign and then the comma from the "dollars" column.
Remember to replace the sample data with your actual data and adjust the column name as needed.
Paella Pan for Two: What Size?
You may want to see also
Frequently asked questions
You can use the replace() method to remove the currency sign from a column in your Pandas dataframe. For example:
```python
df['column_name'] = df['column_name'].str.replace('$', '')
```
You can specify multiple columns to remove the currency sign from by passing a list of column names to the `replace()` method:
```python
cols = ['col1', 'col2', ..., 'colN']
df[cols] = df[cols].replace({'\$': ''}, regex=True)
```
You can remove both the currency sign and commas from a column in your Pandas dataframe by specifying both characters in the `replace()` method:
```python
df['column_name'] = df['column_name'].str.replace('$,', '')
```
You can remove the currency sign from your entire Pandas dataframe by specifying the columns you want to check and then using the `replace()` method with the regex=True argument:
```python
colstocheck = dftest.columns
dftest[colstocheck] = dftest[colstocheck].replace({'\$': ''}, regex=True)
```
To remove the currency sign and other random symbols from your Pandas dataframe, you can use the `replace()` method with the regex=True argument. For example, to remove the currency sign, commas, and percentages from a column:
```python
df['column_name'] = df['column_name'].str.replace('$,%', '')
```